Last Updated | | Ratings | | Unique User Downloads | | Download Rankings |
2020-07-27 (4 months ago)  | | Not yet rated by the users | | Total: 23 | | All time: 10,102 This week: 38 |
|
Description | | Author |
This package is a wrapper to send HTTP requests using Guzzle package.
It provides a fluent interface to create requests, set request parameters, send requests and retrieve responses. Currently it can:
- Send GET, POST, PUT, DELETE, HEAD, CONNECT, PATCH and OPTIONS requests
- Set request cookies
- Set request the user agent from a list of known browser user agents
- Set file uploads
- Get response headers
- Handle redirections | |
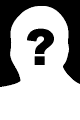 |
|
Innovation award
 Nominee: 4x |
|
Details
Guzwrap, PHP GuzzleHttp Wrapper.
Guzwrap is a wrapper that provides easy helper functions
around PHP popular web client library, GuzzleHttp.
Installation
Make sure that you have composer installed
Composer.
If you don't have Composer run the below command
curl -sS https://getcomposer.org/installer | php
Now, let's install Guzwrap:
composer require ahmard/guzwrap
After installing, require Composer's autoloader in your code:
require 'vendor/autoload.php';
Usage
use Guzwrap\Request;
//simple request
$result = Request::get($url)->exec();
//with authentication
Request::get($url)
->auth('username', 'password')
->exec();
-
get Guzwrap Instance
$instance = Request::getInstance();
//Do something...
-
Request with cookies
Request::get($url)
->withCookie()
//or use cookie file
->withCookieFile($fileLocatiom)
//use cookie session
->withCookieSession($name)
//use array too
->withCookieArray([
'first_name' => 'Jane'
'other_names' => 'Doe'
])->exec();
-
Handle redirects
Request::get($url)
->redirects(function($wrp){
$wrp->max(5);
$wrp->strict();
$wrp->referer('http://goo.gl');
$wrp->protocol('http');
$wrp->trackRedirects();
$wrp->onRedirect(function(){
echo "Redirection detected!";
});
})->exec();
-
Headers
Request::get($url)->header(function($h){
$h->add('hello', 'world');
$h->add('planet', 'earth');
})->exec();
-
Query
Request::get('https://google.com')
->query('q', 'Who is jane doe')
->exec();
-
Post form data
Request::url($url)->post(function($req){
$req->field('first_name', 'Jane');
$req->field('last_name', 'Doe');
})->exec();
//Post with multipart data
Request::url($url)->post(function($req){
$req->field('full_name', 'Jane Doe');
$req->file('avatar', 'C:\jane_doe.jpg');
})->exec();
//Alter file data
Request::url($url)->post(function($req){
$req->field('full_name', 'Jane Doe');
$req->file(function(){
$file->field('avatar');
$file->path('C:\jane_doe.jpg');
$file->name('John_doe.gif');
});
})->exec();
-
UserAgent
We provide custom useragents to help send request easily.
Request::userAgent('chrome');
//Choose specific useragent index from array
Request::userAgent('chrome', '1');
//Choose sub-useragent
Request::userAgent('chrome', '9.1');
List useragents
use Guzwrap\UserAgent;
$userAgents = (new UserAgent())->getAvailable();
|
Applications that use this package |
|
No pages of applications that use this class were specified.
If you know an application of this package, send a message to the author to add a link here.