Last Updated | | Ratings | | Unique User Downloads | | Download Rankings |
2023-08-16 (1 month ago)  | | Not yet rated by the users | | Total: 17 This week: 1 | | All time: 11,045 This week: 102 |
|
Description | | Author Smoren
Contributor
|
This class can manage lists of values like associative arrays.
It provides several functions to change the list of values that the class stores.
Currently, the class can:
- Initialize the list of values with an array
- Check if the list has a given key
- Get the value of a key and return a default if the key is missing
- Set the value of a key with the option to throw an exception if there is a key with the same value
- Remove a value with a given key
- Remove all values of the list Innovation Award
 August 2023
Nominee
Vote |
Arrays are a frequently used data type that PHP developers use in their code.
Often associative arrays are used to store lists of values.
This package provides an alternative class that implements lists of values that can assume default values when retrieving list values that are not set.
It can also prevent setting a list value that is already set.
These features allow to implement more restricted lists of values in PHP.
Manuel Lemos |
| |
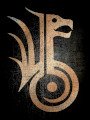 |
|
Innovation award
 Nominee: 14x |
|
Details
yii2-array-storage
????? ??? ???????? ? ?????????? ??????? ? ???????.
????? ???? ???????? ?? ????????, ????????, ? ???????? ??????? ??? ??????
? ?????, ???????? _json_ ? ?????? _ActiveRecord_.
????????? ? ?????? ?? Yii2
composer require smoren/yii2-array-storage
??????? ?????????????
<?php
use Smoren\Yii2\ArrayStorage\Storage;
// ???????? ??????
$data = [
'a' => [
'b1' => [1, 2, 3],
'b2' => 5,
]
];
// ????????????? ?????????
$storage = new Storage($data);
// ????????? ????? ??????? ?????? ?????????
$value = $storage->get();
print_r($value);
/*
Array
(
[a] => Array
(
[b1] => Array
(
[0] => 1
[1] => 2
[2] => 3
)
[b2] => 5
)
)
*/
// ????????? ???????? ?? ?????
$value = $storage->get('a');
print_r($value);
/*
Array
(
[b1] => Array
(
[0] => 1
[1] => 2
[2] => 3
)
[b2] => 5
)
*/
// ????????? ???????? ?? ????? ? ???????? ???????????
$value = $storage->get('a.b1.0');
var_dump($value);
/int(1)/
// ??????? ????????? ???????? ?? ?????????????? ? ????????? ????? ?? ????????? ?? ?????????
$value = $storage->get('a.b3', '???????? ?? ?????????');
var_dump($value);
/string(40) "???????? ?? ?????????"/
// ??????? ????????? ???????? ?? ?????????????? ? ????????? ????? ??? ???????? ?? ?????????
try {
$storage->get('a.b3');
} catch(\yii\base\Exception $e) {
var_dump($e->getMessage());
/string(39) "key 'a.b3' is not exist in user storage"/
}
// ???????? ????????????? ????? ? ???????
var_dump($storage->has('a.b2'));
/bool(true)/
var_dump($storage->has('a.b3'));
/bool(false)/
try {
$storage->has('a.b3', true);
} catch(\yii\base\Exception $e) {
var_dump($e->getMessage());
/string(39) "key 'a.b3' is not exist in user storage"/
}
// ?????????? ???????? ? ?????????
$storage->set('a.new', '???????? ?????? ????????');
print_r($storage->get());
/*
Array
(
[a] => Array
(
[b1] => Array
(
[0] => 1
[1] => 2
[2] => 3
)
[b2] => 5
[new] => ???????? ?????? ????????
)
)
*/
// ?????????? ???????? ? ????????? ? ???????? ??????????
$storage->set('a.new_another', '??? ???? ???????? ?????? ????????', false);
print_r($storage->get());
/*
Array
(
[a] => Array
(
[b1] => Array
(
[0] => 1
[1] => 2
[2] => 3
)
[b2] => 5
[new] => ???????? ?????? ????????
[new_another] => ??? ???? ???????? ?????? ????????
)
)
*/
// ??????? ?????????? ???????? ? ???????? ??????????
try {
$storage->set('a.b2', '??? ???????? ?? ?????????', false);
} catch(\yii\base\Exception $e) {
var_dump($e->getMessage());
/string(43) "key 'a.b2' is already exist in user storage"/
}
print_r($storage->get());
/*
Array
(
[a] => Array
(
[b1] => Array
(
[0] => 1
[1] => 2
[2] => 3
)
[b2] => 5
[new] => ???????? ?????? ????????
[new_another] => ??? ???? ???????? ?????? ????????
)
)
*/
// ???????? ???????? ?? ????????? ?? ?????, ????????? ???????? ?????????? ????????
$removedValue = $storage->remove('a.b1');
print_r($removedValue);
/*
Array
(
[0] => 1
[1] => 2
[2] => 3
)
*/
print_r($storage->get());
/*
Array
(
[a] => Array
(
[b2] => 5
[new] => ???????? ?????? ????????
[new_another] => ??? ???? ???????? ?????? ????????
)
)
*/
// ??????? ???????? ??????????????? ????????
try {
$storage->remove('a.?');
} catch(\yii\base\Exception $e) {
var_dump($e->getMessage());
/string(39) "key 'a.?' is not exist in user storage"/
}
|
Applications that use this package |
|
No pages of applications that use this class were specified.
If you know an application of this package, send a message to the author to add a link here.