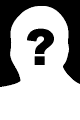
Bodor Bence - 2016-07-25 18:43:57
Hi there.
Just dropping my 2 cents.
If your main goal is optimalization, you should really take it easy on the unnecessary function calls in your script. I mean this:
$ip_data = array();
foreach ($ip_list as $ip) {
// get all records
$records = $db->lookup($ip, \IP2Location\Database::ALL);
array_push($ip_data, array(
'ipaddr' => $records['ipAddress'],
'countryCode' => $records['countryCode'],
'regionName' => $records['regionName'],
'cityName' => $records['cityName'],
'lat' => $records['latitude'],
'long' => $records['longitude']
));
}
Is the same as this:
$ip_data = array();
foreach ($ip_list as $ip) {
// get all records
$records = $db->lookup($ip, \IP2Location\Database::ALL);
$ip_data[] = array(
'ipaddr' => $records['ipAddress'],
'countryCode' => $records['countryCode'],
'regionName' => $records['regionName'],
'cityName' => $records['cityName'],
'lat' => $records['latitude'],
'long' => $records['longitude']
);
}
Except the latter is 3 to 4 times faster (on a 100k list), because it doesn`t use a function call each time to add something to an array.
Also, array_key_exists($key, $search_array) is in most cases the same as isset($search_array[$key]) (not going into the edge cases right now) which is 2 times faster than array_key_exists.
Same goes for array_merge. If all you want to do is merge 2 simple arrays and you are going for speed, don`t be lazy to write a simple foreach to add in the values manually like in my first suggestion in this reply.
And as somebody before me mentioned, you should really test your script against the "slower" method in a real life exaplme too, before calling it faster.
P.S.: Can I make a code block in my reply somehow? Cause I can`t find any way to do it, or any description about it anywhere. I kinda find that strange on a site about scripting...