|
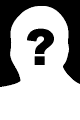 Peter Barney - 2005-07-28 00:24:14
I have gotten test_personalized_bulk_mail.php to run successfully, and also have gotten test_smtp.php to work using an Exchange server which requires SASL and NTML authentication.
However, I don't understand how to send bulk email using smtp with these classes. How do I combine the two to get them to work together?
Perhaps it would be better to explain my goal: I want to send email messages as efficiently as possible using only PHP. I can either send them to an Exchange server for delivery or would it be better to just use my web host's sendmail?
Please help!
Peter
 Manuel Lemos - 2005-07-29 04:21:27 - In reply to message 1 from Peter Barney
To use the SMTP delivery method, you need to use the smtp_message_class sub-class instead of the email_message_class.
That sub-class is specialized in delivering the messages via a SMTP server, instead of the mail() function used by the base class.
Anyway, queuing messages via SMTP is usually not faster than using sendmail.
What happens is that when you use the sendmail program you can inject messages directly in the local mailer queue.
When you use SMTP to relay the messages, you are injecting messages in the local mailer queue indirectly, so you have extra overhead communicating with the mail server via a TCP connection.
Anyway, usually the mail() function uses sendmail on Unix/Linux and SMTP on Windows because those are the most common options that are available, not necessarily the best.
If your site is hosted on a Windows machine with Exchange locally installed, you can use the pickup_message_class that is much faster than connecting to SMTP because it drops messages directly in the Exchange queue folders.
If your site is hosted on a Unix/Linux machine, you can use sendmail_message_class with special options that make it accept messages in its queue.
The bulk mail examples call the class SetBulkMail() that takes care of setting the right options to optimize for bulk mail deliveries regardless of the delivery sub-class that you choose.
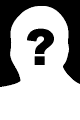 Preseo - 2005-08-17 02:54:03 - In reply to message 2 from Manuel Lemos
Hello,
I have a mySQL database of 20K email addresses... Currently I am using the php mail function but it crashes mySQL server.
Currently I have it coded like this
$QUERY = "SELECT email FROM table";
while ($ROW = MYSQL_FETCH_ARRAY($QUERY))
{
$HTML_MESSAGE = "<HTML>....</HTML>";
mail($ROW[email],$HTML_MESSAGE); //CRASHES SQL SERVER
}
WHICH ONE OF YOUR PROCEDURES DO YOU RECOMMEND? WILL I HAVE TO CUE 20K BY SENDING 1000 emails and then SENDING ANOTHER 1000 after a few MINUTES OR SO?
I tested test_pickup_message.php and test_personalized_bulk_mail.php examples and it worked on my server (I didn't apply my situation to it, I merely changed the email address to mine in the samples)
PLEASE HELP... THANKS IN ADVANCE
 Manuel Lemos - 2005-08-17 03:27:43 - In reply to message 3 from Preseo
If the pickup example worked for you, I assume you use a Windows environment on which the pickup_message_class can be used. In that case that is the ideal class for bulk mailing under Windows platforms.
Just watch your disk space because what the class does is to drop messages in a folder but your MTA (Micrsoft Exchange I suppose) will take some time to deliver all the queued messages. While the messages are not delivered, they remain in the queue occupying disk space.
Depending on the bandwidth available in your network, tens of thousands of messages may take hours to deliver.
As for crashing your MySQL server, that is an odd thing. Did the server run out of memory? I don't know how your mailing loop may have caused that alone.
Anyway, if you can avoid personalizing your mailing message body, do not hesitate. In that case you can tell the class to cache your message body to avoid message rebuilding memory usage and queueing overhead.
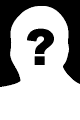 Preseo - 2005-08-17 04:03:29 - In reply to message 4 from Manuel Lemos
Thank you for your quick response.
In summary, will it be like this?
///////////////////////
$QUERY = "SELECT email FROM table";
while ($ROW = MYSQL_FETCH_ARRAY($QUERY))
{
$HTML_MESSAGE = "<HTML>....</HTML>";
//SEND AN EMAIL USING THE pickup_message_class
}
////////////////////////
1. ALL MESSAGES WILL BE THE SAME. IT WILL NOT BE CUSTOMIZED. SO I BELIEVE THERE IS A FLAG THAT NEEDS TO BE SET CORRECT? CASHING THE MEMORY... DOES THAT PREVENT THE DISK SPACE ISSURE YOU DISCUSSED IN PARAGRAPH TWO
2. SHOULD I SEND 1000 AT A TIME OR SHOULD I LOAD ALL AT ONCE?
THANK IN ADVANCE SIR!
 Manuel Lemos - 2005-08-17 05:14:34 - In reply to message 5 from Preseo
Yes, that is more or less like that.
If your messages are not personalized, I suggest that you just set one email message object outside of the database query result traversal loop as you see in the bulk mail examples. You can set the message body also outside the loop, and only set the To: header and call the class Send function inside the loop.
To tell the class to cache the message body just set the class variable cache_body to 1.
The memory usage was just a guess for crashing the server. I don't know if it is the real reason.
The disk space issue can be avoided by checking it once in a while using the PHP disk_free_space() function on the actual disk partition where the queue is stored.
If it is below an acceptable threshold, use the sleep() function to make your script rest for a period and then check the disk space again. If disk space is ok, resume your deliveries, otherwise wait another period, and so on.
You can make the disk space check on every 100 deliveries, but you do not need to exit your script to make that check. You can do it all in a single script.
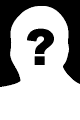 Preseo - 2005-08-20 20:50:06 - In reply to message 6 from Manuel Lemos
One last question...
How would you enable html in the test_pickup_message.php class?
Thanks
You have been much help
 Manuel Lemos - 2005-08-21 01:12:24 - In reply to message 7 from Preseo
To send HTML messages, there is an extensive explanation in Usage section of the main documentation file of the package documentation/email_message_class.html , including details on how to embed images in HTML messages and add alternative text parts to the HTML parts.
Anyway, the easiest way to get started is to start with the test_simple_html_mail_message.php example and change where it says:
$email_message=new email_message_class;
to
$email_message=new pickup_message_class;
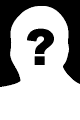 Preseo - 2005-11-19 18:13:54 - In reply to message 8 from Manuel Lemos
I just recently switch servers from Windows to Linux. I am using cpanel with the new server. The mail program was working fine on old server but now i get this error.
Error: it was not specified the mailroot directory path
PLEASE HELP
THANKS IN ADVANCE
 Manuel Lemos - 2005-11-19 19:16:56 - In reply to message 9 from Preseo
The pickup folder delivery method is only available on Windows because it drops messages in the Microsoft Exchange or IIS queue folder.
Under Linux use the sendmail_message_class class or even email_message_class base class.
$email_message=new sendmail_message_class;
|