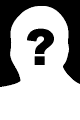
achilles - 2010-09-20 15:29:16
For zipping a single file, this works well:
<?php
include_once("../classes/CreateZipFile.inc.php");
$createZip = new createZipFile;
$createZip -> addDirectory("testy/");
$fileContents = file_get_contents("../testDirectory/Test1.txt");
$createZip -> addFile($fileContents, "testy/Test1.txt");
$fileName = "testone.zip";
$fd = fopen ($fileName, "wb");
$out = fwrite ($fd, $createZip -> getZippedfile());
fclose ($fd);
$createZip -> forceDownload($fileName);
@unlink($fileName);
?>
But for multiple files from a directory, this produces a zipped file, containing the expected directory 'testy' - but 'testy' has no files in it (it should have Test1.txt and Test2.txt):
<?php
$fileToZip = "Test1.txt";
$directoryToZip ="../testDirectory";
include_once("../classes/CreateZipFile.inc.php");
$createZipFile=new CreateZipFile;
$outputDir ="testy/";
$zipName = "test.zip";
$createZipFile->zipDirectory($directoryToZip,$outputDir);
$fd=fopen($zipName, "wb");
$out=fwrite($fd,$createZipFile->getZippedfile());
fclose($fd);
$createZipFile->forceDownload($zipName);
@unlink($zipName);
?>
I am guessing that the problem ins with $fileToZip, since I have not figured what it does inside the class, and I am guessing that in turn affects public function addFile - though I don't see how.
I'd be grateful for a correction to my code - and, if you have time, a brief note on how the correction makes the class work correctly.