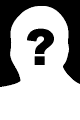
Wassermann Tobias - 2007-06-20 06:26:48
Hi,
another possibility to resolve the old php-code-in-image-problem is the usage of fileinfo and the imagecreatefromXXX-functions().
Fileinfo (get it from http://pecl.php.net/package/Fileinfo) is a libmagic related PHP-Extension. It will determine the filetype through content-analysis. The only thing you need is a mime.magic-file (eg the apache will come with one). So the initialisation is simple:
$info = new finfo("/www/mime.magic");
To check a file, you'll call:
$ftype = $info->file($_FILES["filename"]["tmp_name"]);
[Hint: Here I'll use the OOP-Version of fileinfo, there are also "classical" functions available. Look at http://www.php.net/manual/en/ref.fileinfo.php]
$ftype now contains the mime-type, such as image/gif, text/plain, application/octet-stream or whatever. Now the second step to remove the PHP code from a image is very simple: Use the imagecreatefrom-functions.
If you have - for example - a gif image with PHP code in it, you can use imagecreatefromgif() to create another gif-file. You can create the second one with the first one as base, then convert it to a png and this one back to gif:
function gif_info($filename)
{
$fp= fopen($filename,'rb');
$result= fread($fp,13);
$file['signatur'] = substr($result,0,3);
$file['version'] = substr($result,3,3);
$file['width'] = ord(substr($result,6,1))+ord(substr($result,7,1))*256;
$file['height'] = ord(substr($result,8,1))+ord(substr($result,9,1))*256;
$file['flag'] = ord(substr($result,10,1))>>7;
$file['trans_red'] = ord(substr($result,ord(substr($result,11))*3,1));
$file['trans_green'] = ord(substr($result,ord(substr($result,11))*3+1,1));
$file['trans_blue'] = ord(substr($result,ord(substr($result,11))*3+2,1));
fclose($fp);
return $file;
}
if($ftype=="image/gif")
{
$nam = tempnam("/tmp", "img");
$img = imagecreatefromgif($_FILES["filename"]["tmp_name"]);
$gifdata = gif_info($_FILES["filename"]["tmp_name"]);
imagepng($img, $nam);
$img = imagecreatefrompng($nam);
if($gifdata["version"] == "89a")
{
imagecolortransparent($img, imagecolorallocate($img, $gifdata['trans_red'], $gifdata['trans_green'], $gifdata['trans_blue']));
}
imagegif($img, "uploads/".$_FILES["filename"]["name"]);
unlink($nam);
}
Now the code is removed from the newly stored file. The if($gifdata["version"] == "89a") ensures transparency from origin file is also included in the new gif.
Bye
Tobias