Author: Stefan Kientzler
Updated on: 2023-08-30
Posted on: 2023-08-30
Viewers: 61 (August 2023)
Package: PHP Google Contacts API
Read this short tutorial to learn how to use this package to retrieve, change, or delete contacts of a given user with a Google account.
This package can be installed traditionally by downloading the package files from the package download tab or using the modern form, using the PHP Composer tool by following the instructions in the package page download tab.

Overview
To manage Google contacts using the Google Person API, there are three steps:
- Create a Google project in the Google developer console to get an API key and OAuth 2.0 client data
- Login with the desired Google account
- Sending HTTP requests to read, write, or delete contact data
All that is needed to implement these steps is included in this package, which only uses the standard PHP cURL library and has no further dependencies on any other 3rd party libraries or packages.
1. Create a Google Project in the Google Developer Console
The first step is to create and set up a new project in the Google developer console that you can access by going to the page https://console.cloud.google.com/.
- Create a project with your preferred name
- Enable the Google People API
-
Create Credentials
- Credential Type
- Select API: People API
- Check the 'User data' option
- OAuth Consent Screen
- Enter the App information and the Developer's contact information
- Scopes
- Type
contacts
in the filter and then set the check to
[x] .../auth/contacts
[x] .../auth/contacts.readonly
[x] .../auth/contacts.other.readonly.
- Type
- OAuth Client ID
- Select Application type: Web application
- Enter a name for your client (the name of your OAuth 2.0 client is only used to identify the client in the console and will not be shown to end users)
- Authorized redirect URIs
- For the live system, enter the URI to the script that receives an authentication code after login.
- For local development and using the example code, enter
http://localhost/<path-to-package>/GoogleOauth2Callback.php
- Your Credentials
Download the created credential information in JSON format. For later access, this is always available on the credentials page.Save the credentials in
secrets/google_secrets.json
in the package directory.
- Credential Type
-
Add Test user(s)
While developing and testing your app, you must add the users that have access as 'test user' (even your own account has to be added):- Join 'APIs & services -> OAuth consent screen' on the left in the Google console
- scroll to the section 'Test users'
- Add the users you want to access to your web application (including your own account).
2. Login With the Desired Google Account
To send requests to the Google People API, a login with OAuth2 authentication to the desired Google account with the needed scope is required.
The code flow for the login, authentication, using the API, and refresh access token
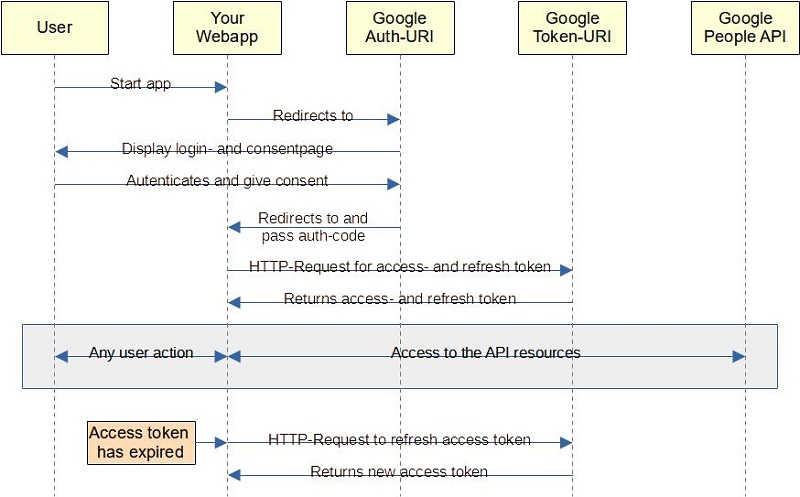
- The user starts the Web application.
- The application builds a Google auth URL using the client ID and specifies the needed scope.
The browser has to be redirected to this URL. - The Google authentication URL shows a login page where the user
- has to enter/select the Google account and log in
- needs to give consent to the requested scope
- The user authenticates and gives consent.
- After a successful login and consent, the browser is redirected to the configured redirect-URI of your web application and passes an authentication code.
- The Web application sends an HTTP request to the Google token URI using the received code, the client ID, and the client secret to fetch the access and refresh tokens.
- On success, the web application gets the tokens and saves both.
- Access the API resources.
- After the access token has expired, the Web application can request a new access token using the refresh token (or can perform a new login - which will undoubtedly annoy the user...).
- The application can decide (or ask the user...) how long the refresh token is saved (keep login until...).
Implementation using the package
At least 2 PHP files are needed to realize this login:
- Build the authentication URI and redirect to the respective page
- The configured redirect-URI to receive the auth-code
The prerequisite is an existing OAuth client configuration in the secrets/google_secrets.json
file.
After the user has logged in and given consent to access the requested scope, the configured redirect-URI for the used OAuth client is called, and the the auth code is passed in the URI param. If the configured redirect URI is unavailable, the authentication fails and the generated authentication code is invalid.
Build the Authentication URI and Redirect to that Page
$oSecrets = new GSecrets();
$oClient = new GClient();
$oClient->setOAuthClient($oSecrets->getClientSecrets());
$oClient->addScope(GContacts::CONTACTS);
$oClient->addScope(GContacts::CONTACTS_OTHER_READONLY);
$strAuthURL = $oClient->buildAuthURL();
header('Location: ' . filter_var($strAuthURL, FILTER_SANITIZE_URL));
Note:
If the OAuth client configuration is available on another location and in another file thansecrets/google_secrets.json
, use thesetSecretsPath()
andsetSecretsFilename()
methods of theGSecrets()
class.In any case, it should be ensured that this file cannot be accessed from outside since it contains the client secret in addition to the IDs and URIs, which are required to query the tokens.
Receive auth code and request tokens
$oSecrets = new GSecrets();
$oClient = new GClient();
$oClient->setOAuthClient($oSecrets->getClientSecrets());
if ($oClient->fetchTokens($_GET['code'])) {
$oSecrets->saveRefreshToken($oClient->getRefreshToken());
$oSecrets->saveAccessToken($oClient->getAccessToken());
header('Location: ./ContactList.php');
}
Note:
After the tokens have been received and saved, the application can start at his entry point.
In the example, the contact list is displayed.
3. Sending HTTP Requests to Read, Write or Delete Contact Data
Several API resources can be accessed after a successful login and saved access token.
Each access to an API resource requires a instance of the GClient class initialized with a valid access token. Furthermore, the expiration date of the access token has to be checked. If the token has expired, first a new token have to be requested before continuing.
$oSecrets = new GSecrets();
$oClient = new GClient();
$oClient->setAccessToken($oSecrets->getAccessToken());
if ($oClient->isAccessTokenExpired()) {
// try to refresh the accesstoken
$strRefreshToken = $oSecrets->getRefreshToken();
if (empty($strRefreshToken)) {
// no refresh token available - redirect to google login
header('Location: ./GoogleLogin.php');
exit;
}
$oClient->setOAuthClient($oSecrets->getClientSecrets());
$oSecrets->saveAccessToken($oClient->refreshAccessToken($strRefreshToken));
}
Use the created GClient
instance to access the API resource.
- class
GContacts
for access to the contacts of the user - class
GContactGroups
for access to the contact groups of the user
$oContacts = new GContacts($oClient);
$oContacts->addPersonFields(GContacts::DEF_LIST_PERSON_FIELDS);
$oContacts->setPageSize(50);
$aContactList = $oContacts->search($strSearch);
If one of the methods that call the Google API fails (if it returns false
), the last
response code and further information can be retrieved through the following methods of the GClient
instance.
-
$oClient->getLastResponseCode()
-
$oClient->getLastError()
-
$oClient->getLastStatus()
4. How to Download and Install the PHP Google Contacts API Package
You need to be a registered user or login to post a comment
Login Immediately with your account on:
Comments:
No comments were submitted yet.